Understanding Telegram Programming Code for Developers
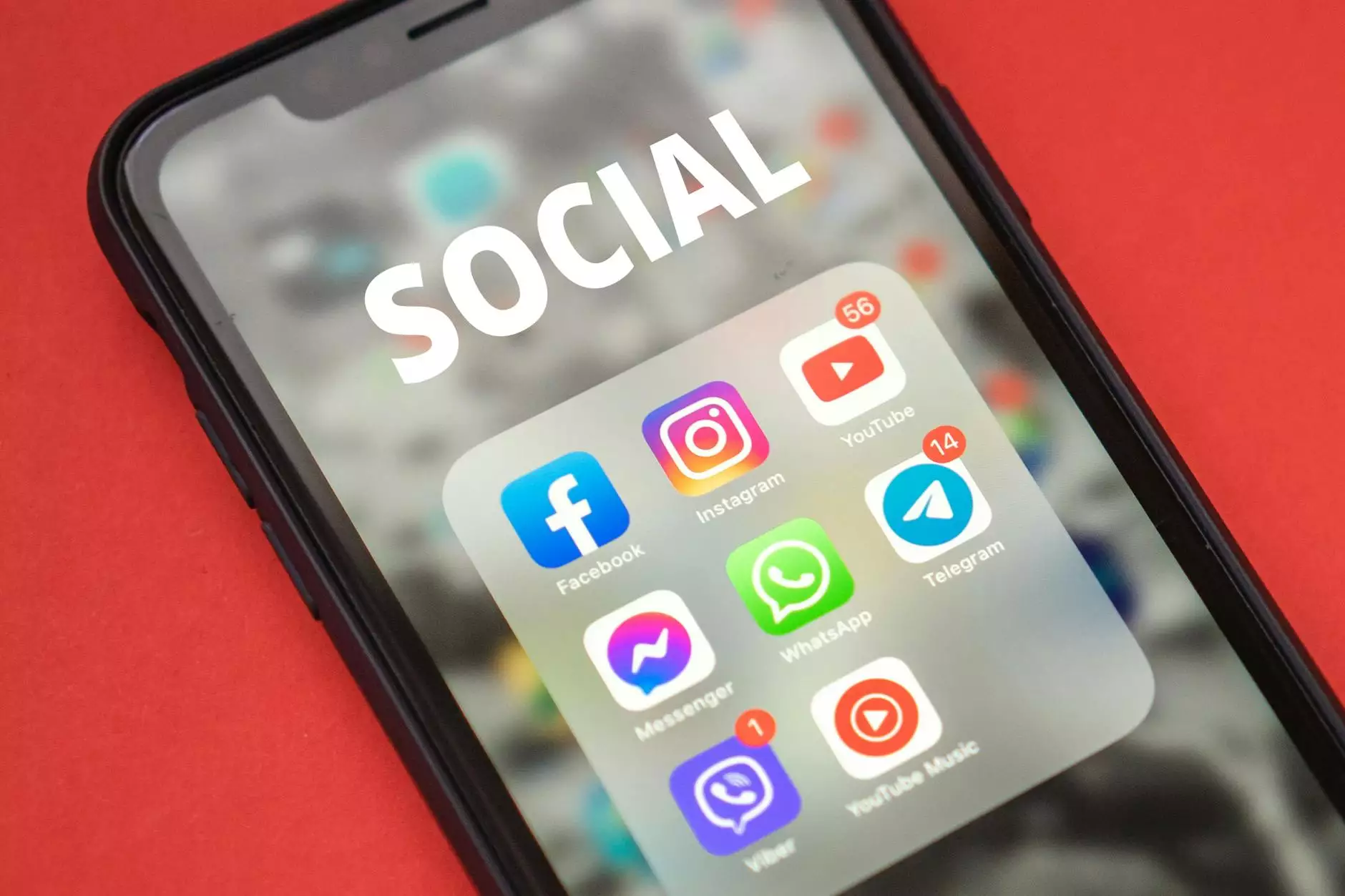
In the modern world of instant messaging and communication, Telegram stands out as one of the most powerful platforms available. Its robust features and extensibility through APIs allow developers to create innovative applications and bots. This article will delve into the various aspects of telegram programming code, exploring languages, libraries, and practical tips for developing effective Telegram bots.
What is Telegram?
Telegram is a cloud-based messaging app that prioritizes speed and security, boasting over 500 million active users worldwide. Unlike many other messaging apps, it provides an open API that allows developers to create their own bots and integrate with the platform, enhancing user experience and workflow.
The Importance of Telegram Programming Code
Understanding telegram programming code is crucial for developers who wish to leverage the Telegram API effectively. Whether you're a seasoned programmer or just starting, being familiar with Telegram's capabilities can open up a world of opportunities:
- Automate tasks through bots
- Enhance user engagement and interaction
- Integrate with other applications
- Deliver content and notifications automatically
Programming Languages for Telegram Bot Development
Below, we will discuss some of the most popular programming languages used to create Telegram bots, detailing the libraries available for each language.
1. Python
Python is one of the most favored languages for creating Telegram bots due to its simplicity and readability. The python-telegram-bot library offers a convenient way to interact with the Telegram API. Here is a basic example of how to create a Telegram bot using Python:
import logging from telegram import Update from telegram.ext import Updater, CommandHandler, CallbackContext # Enable logging logging.basicConfig(format='%(asctime)s - %(name)s - %(levelname)s - %(message)s', level=logging.INFO) logger = logging.getLogger(__name__) # Define a command handler def start(update: Update, context: CallbackContext) -> None: update.message.reply_text('Hello! I am your bot!') def main() -> None: # Create the Updater and pass it your bot's token updater = Updater("YOUR_TOKEN") # Get the dispatcher to register handlers dispatcher = updater.dispatcher # Register the command handler dispatcher.add_handler(CommandHandler("start", start)) # Start polling for updates updater.start_polling() updater.idle() if __name__ == '__main__': main()2. JavaScript (Node.js)
JavaScript is increasingly popular for backend programming through Node.js. The node-telegram-bot-api library simplifies creating Telegram bots with JavaScript. Here’s a simple example:
const TelegramBot = require('node-telegram-bot-api'); // Create a bot that uses 'polling' to fetch new updates const bot = new TelegramBot('YOUR_TOKEN', {polling: true}); // Listen for the '/start' command bot.onText(/\/start/, (msg) => { const chatId = msg.chat.id; bot.sendMessage(chatId, 'Welcome! I am your JavaScript Telegram bot!'); });3. PHP
PHP remains a robust choice for server-side scripting and can be effectively used to create Telegram bots with libraries such as php-telegram-bot. Here is a basic example:
require 'vendor/autoload.php'; use Longman\TelegramBot\Telegram; // Create instance $telegram = new Telegram('YOUR_TOKEN', 'YOUR_BOT_NAME'); // Handle incoming messages $telegram->handle();4. Java
Java is a widely-used language in enterprise environments. The TelegramBots library allows for straightforward bot creation. Below is an example:
import org.telegram.telegrambots.bots.TelegramLongPollingBot; import org.telegram.telegrambots.meta.exceptions.TelegramApiException; public class MyTelegramBot extends TelegramLongPollingBot { @Override public void onUpdateReceived(Update update) { // Process incoming messages here } @Override public String getBotUsername() { return "YOUR_BOT_NAME"; } @Override public String getBotToken() { return "YOUR_TOKEN"; } }5. C#
C# developers can create Telegram bots using libraries such as Telegram.Bot. Here’s an example code snippet:
using Telegram.Bot; using Telegram.Bot.Args; class Program { static void Main(string[] args) { var botClient = new TelegramBotClient("YOUR_TOKEN"); botClient.OnMessage += Bot_OnMessage; botClient.StartReceiving(); } private static void Bot_OnMessage(object sender, MessageEventArgs e) { if (e.Message.Text != null) { Console.WriteLine($"Received a message from {e.Message.Chat.Id}: {e.Message.Text}"); } } }6. Ruby
Ruby enthusiasts can utilize the telegram-bot-ruby gem to manage bot development easily. Below is a sample implementation:
require 'telegram/bot' Telegram::Bot::Client.run('YOUR_TOKEN') do |bot| bot.listen do |message| case message.text when '/start' bot.api.send_message(chat_id: message.chat.id, text: "Hello! I am a Ruby bot!") end end endKey Features of Telegram API
The Telegram API offers numerous features that developers can leverage when programming Telegram bots:
- Message Types: Bots can send and receive various message types including text, images, and video.
- Inline Mode: Users can interact with bots directly in chat without adding them to a group.
- Webhooks: Bots can utilize webhooks for real-time communication.
- User Permissions: Bots can manage group conversations and moderate content based on set permissions.
Best Practices for Developing Telegram Bots
When diving into telegram programming code, following best practices can save time and improve the quality of your bots:
- Understand the API Documentation: Familiarize yourself with the official Telegram Bot API Documentation to utilize its features effectively.
- Implement Error Handling: Ensure robust error handling to account for unexpected scenarios in communication and data processing.
- Optimize Performance: Minimize response times by using efficient data processing and asynchronous calls where applicable.
- Maintain User Privacy: Adhere to privacy standards, ensuring that personal data is securely handled.
- Regular Updates: Keep your bot updated with the latest features and security enhancements provided by Telegram.
Conclusion
Learning and mastering telegram programming code opens up a plethora of opportunities within the digital space. As more businesses and users engage with Telegram, the demand for sophisticated and functional bots will only continue to grow. By leveraging the right programming languages and adhering to best practices, you can contribute significantly to this community, enhancing user engagement while automating essential tasks. Start your journey today and create bots that deliver outstanding value to users around the world!